Creating a 3D Game from Scratch with C++
Creating a 3D Game from Scratch with C++
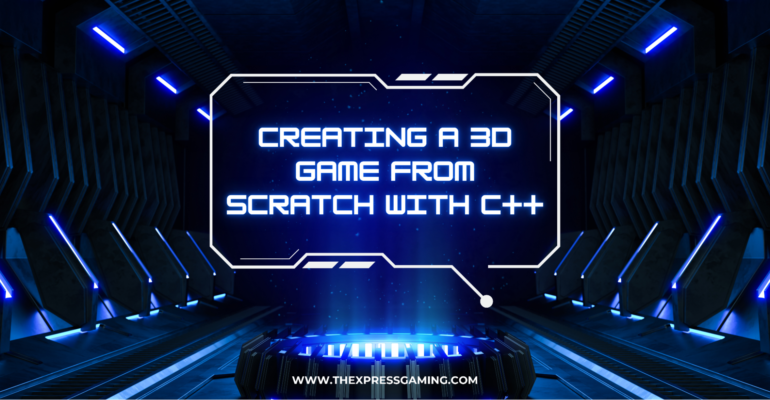
Contents
- 1 What is 3D Game Development?
- 2 Why Choose C++ in 3D Game Development?
- 3 How to Build a 3D Game with C++?
- 3.1 Step 1: Understand the Basics of Game Development
- 3.2 Step 2: Choose the Right Game Engine
- 3.3 Step 3: Master C++ for Game Development
- 3.4 Step 4: Learn Mathematics for Game Development
- 3.5 Step 5: Explore Graphics Programming
- 3.6 Step 6: Specialize in Game Development Areas
- 3.7 Step 7: Practice with Game Projects
- 3.8 Step 8: Stay Updated with Industry Trends
- 3.9 Step 9: Build a Portfolio
- 3.10 Step 10: Test and Debug Your Game
- 4 Winding Up
Are you dreaming of creating your own 3D game and wondering which programming language is best suited for game development?
The answer is— C++!
C++ is a powerhouse in the gaming industry, especially for 3D games. Its ability to provide unmatched performance and fine-tuned control over game systems makes it the go-to language for many top developers.
Is it the only language used for AAA 3D games?
Not exactly—but its role is crucial.
In this blog, we’ll discuss why C++ is so widely used in 3D game development, how it gives developers a competitive edge, and why it should be your language of choice if you plan to bring your game ideas to life.
Let’s jump in!
What is 3D Game Development?
3D game development involves creating video games with three-dimensional environments, objects, and characters that can be interacted with in a 3D space. Unlike 2D games, which have flat visuals, 3D games provide a sense of depth and allow players to explore the game world in multiple directions.
In 3D game development, you’ll design and animate models, create realistic physics, and implement lighting to bring the world to life. You also need to handle the player’s interaction with the environment and objects in that 3D space, making the gameplay experience immersive.
Here are some features of 3D game development.
- Immersive Environments: 3D games simulate realistic environments, often including detailed textures, realistic lighting, and dynamic weather effects. This creates a deep sense of immersion. Spatial design allows players to explore vast, open worlds or intricately designed levels, enhancing their overall experience.
- Realistic Physics and Animations: Physics in 3D games mimics the real world, ensuring that objects move, collide, or interact as they would in reality. For example, gravity affects objects realistically, and explosions can scatter debris. Animations, such as character movements or environmental interactions, are crafted to look fluid and believable, adding to the game’s authenticity.
- Three-Dimensional Interaction: In 3D games, players interact with the environment in multiple dimensions, such as moving forward, backward, sideways, or upward. This depth allows for more complex gameplay mechanics, like climbing, flying, or exploring underwater terrains. Players can also interact with objects or characters from various angles, enriching their engagement.
- AI Behavior and Pathfinding: Advanced AI systems in 3D games create dynamic and responsive behavior for non-player characters (NPCs). These characters can adapt to player actions, follow intricate paths, and exhibit lifelike reactions. Pathfinding ensures NPCs can navigate complex terrains or obstacles effectively, making their behavior seem more intelligent and natural.
- Enhanced Visual Fidelity: 3D games utilize high-quality graphics, including realistic textures, lighting, and shadows, to create stunning visuals. Advanced rendering techniques, such as ray tracing, enhance realism by simulating light behavior. These visual elements contribute significantly to the game’s aesthetic appeal and atmosphere.
- Multiplatform Capabilities: Modern 3D games are often designed to run on multiple platforms, such as PCs, consoles, and mobile devices. Developers optimize the game’s performance to ensure consistent quality across various hardware configurations. This flexibility allows a broader audience to enjoy the game, regardless of their device.
These foundational concepts are essential for building immersive 3D games and overcoming the technical challenges of game development. Do you want to discover why C++ is the go-to language for creating high-performance, visually stunning 3D games?
Why Choose C++ in 3D Game Development?
C++ has long been a favorite language for 3D game development due to its unmatched performance and flexibility. It allows developers to create high-performance games while maintaining control over hardware and memory management, making it especially suited for the demanding requirements of 3D games.
Here are the key reasons why C++ stands out in 3D game development.
- High Performance: C++ allows developers to interact directly with hardware, optimizing performance by minimizing latency and maximizing speed. This is crucial for 3D games, where smooth graphics and gameplay are essential.
- Widely Supported: Major game engines like Unreal Engine and Godot use C++ for scripting, ensuring robust tools for game developers. Even though Unity uses C# for scripting, it still relies on C++ in its runtime, highlighting the language’s widespread usage in the industry.
- Low-Level Control: C++ allows developers to manage memory and system resources, offering better optimization opportunities for handling complex 3D environments and game mechanics. This control is vital for games that require heavy computations, such as physics simulations and AI behaviors in 3D worlds.
- Cross-Platform Development: With C++, you can develop games across multiple platforms, including Windows, Mac, Linux, iOS, and Android, ensuring your 3D game reaches a broad audience. This is essential for reaching the global gaming community.
- Speed Advantage: In performance tests, C++ consistently outperforms other languages like Java, C#, and Python. For example, in a binary-tree benchmark, C++ completed the task in just 1,129 milliseconds, far outpacing its competitors.
- Industry Standard: C++ is used by some of the biggest names in the gaming industry, including AAA game studios. Its widespread use in high-end games, from “The Witcher 3” to “Fortnite,” speaks to its capacity to handle the most demanding 3D environments and game features.
- Real-Time Performance: C++ excels in real-time systems, a crucial aspect of 3D game development that requires real-time rendering and interaction. The language’s ability to handle simultaneous processes without lag is essential for real-time 3D rendering.
C++ is a top choice for 3D game developers due to its performance and control over game systems. Now that we’ve explored why C++ is ideal for 3D game development, let’s discuss how to create one!
How to Build a 3D Game with C++?
Creating a 3D game with C++ is a journey that combines technical expertise, creativity, and problem-solving. This guide walks you through every major step, from understanding the basics of game development to optimizing your finished game for distribution. Each step is designed to help you build a robust foundation and grow your skills in game creation.
Here are the steps of creating a 3D game from scratch using C++.
Step 1: Understand the Basics of Game Development
Game development is creating interactive experiences through software, and understanding the fundamental concepts is essential to building your game development skills.
Hence, before diving into coding, it’s important to learn the key building blocks of every game development, such as the game loop, physics simulation, rendering, and input handling. These elements combine to create an immersive and responsive gaming experience for players.
- Game Loop: The game loop is the core structure of every game. The repetitive cycle updates the game state, processes user input, and renders the game world, ensuring the game runs continuously.
Here’s a simple C++ game loop to explain the core structure of game development.
#include <iostream> #include <chrono> #include <thread> void ProcessInput() { // Handle player inputs std::cout << “Processing input…\n”; } void UpdateGame(float deltaTime) { // Update game logic std::cout << “Updating game (deltaTime: ” << deltaTime << ” seconds)…\n”; } void RenderFrame() { // Render graphics std::cout << “Rendering frame…\n”; } int main() { const int FPS = 60; const float frameDuration = 1.0f / FPS; while (true) { auto start = std::chrono::high_resolution_clock::now(); ProcessInput(); UpdateGame(frameDuration); RenderFrame(); auto end = std::chrono::high_resolution_clock::now(); std::chrono::duration<float> elapsed = end – start; // Ensure consistent frame rate std::this_thread::sleep_for(std::chrono::duration<float>(frameDuration – elapsed.count())); } return 0; } |
- Rendering: It involves drawing graphics on the screen and creating visual elements like textures, models, and animations. It also includes advanced techniques for realistic lighting, shadows, and special effects.
- Physics: Many games simulate physical interactions like gravity, object collisions, and movement. Basic physics knowledge is essential to make your game feel realistic.
- Input Handling: Input from the player, whether through the keyboard, mouse, or controller, is an integral part of the gaming experience. Efficient input handling ensures smooth and responsive gameplay.
Here’s an example of simple character movement in Unreal Engine using C++.
void AMyCharacter::MoveForward(float Value) { if ((Controller != nullptr) && (Value != 0.0f)) { const FRotator Rotation = Controller->GetControlRotation(); const FRotator YawRotation(0, Rotation.Yaw, 0); const FVector Direction = FRotationMatrix(YawRotation).GetUnitAxis(EAxis::X); AddMovementInput(Direction, Value); } } |
Step 2: Choose the Right Game Engine
Game engines provide the necessary tools and libraries that streamline the development process, from rendering to physics simulation. Choosing the right engine is crucial, depending on the scale, complexity, and platform you’re targeting. There are several widely used engines for creating 3D games.
- Unreal Engine: Unreal Engine is a leading tool for creating visually stunning games. It uses C++ and offers Blueprints, a visual scripting tool, to create games without extensive coding. Due to its advanced rendering capabilities, Unreal is ideal for high-end 3D games and AAA projects.
- Godot: Godot is a lightweight, open-source engine ideal for smaller 3D projects. While its 3D engine has improved over time, it still lags behind engines like Unreal or Unity (which primarily uses C#) for performance-heavy 3D games.
- CryEngine: CryEngine is known for its high-quality visuals but has a smaller community than Unreal and Unity. It’s typically used for high-end, visually immersive games but is less commonly used in the industry today due to its complexity and smaller developer base.
Unreal Engine is the most recommended for beginners because of its extensive resources, tutorials, and community support.
Step 3: Master C++ for Game Development
C++ is one of the most powerful and widely used languages in game development, especially for performance-intensive applications. Mastering C++ for game development requires understanding the language’s syntax, object-oriented principles, and key aspects such as memory management, performance optimization, and data structures.
- Memory Management: C++ gives developers full control over memory, which is crucial for creating high-performance games. Using smart pointers like std::unique_ptr and std::shared_ptr helps manage memory allocation, preventing memory leaks and ensuring safer memory handling.
Here’s how smart pointers are important in C++ game development.
#include <iostream> #include <memory> class GameEntity { public: GameEntity(const std::string& name) : name(name) { std::cout << “Entity ” << name << ” created.\n”; } ~GameEntity() { std::cout << “Entity ” << name << ” destroyed.\n”; } private: std::string name; }; int main() { { std::shared_ptr<GameEntity> player = std::make_shared<GameEntity>(“Player”); std::weak_ptr<GameEntity> weakPlayer = player; // Weak reference to prevent cyclic dependency } // Automatically cleans up the memory here return 0; } |
- Object-Oriented Programming (OOP): OOP is essential for creating organized and efficient game code. Concepts like inheritance, polymorphism, and encapsulation help you structure game logic, making managing complex systems and behaviors easier.
- Performance Optimization: Performance is crucial in game development. Understanding how to optimize your code using profiling tools and techniques, such as algorithm optimization and resource management, ensures that your game runs smoothly, even with complex scenes and high graphical fidelity.
- Data Structures and Algorithms (DSA): Knowledge of DSA is vital for solving complex problems efficiently. This is especially useful for handling AI behavior, pathfinding, and game physics calculations.
A deep understanding of these concepts is crucial when creating your custom game engine or addressing performance bottlenecks.
Step 4: Learn Mathematics for Game Development
Mathematics is the foundation of many game development concepts, especially in 3D rendering, physics, and animation. Understanding key math concepts is essential for creating realistic and interactive game mechanics.
- Linear Algebra: Linear algebra is used to manipulate objects in 3D space, such as rotating, scaling, and translating objects. It is the core of transforming coordinates and is used extensively in graphics programming.
Here’s a C++ example of basic 3D transformations.
#include <iostream> #include <glm/glm.hpp> #include <glm/gtc/matrix_transform.hpp> int main() { glm::vec3 position(0.0f, 0.0f, 0.0f); glm::vec3 direction(1.0f, 0.0f, 0.0f); glm::mat4 translationMatrix = glm::translate(glm::mat4(1.0f), glm::vec3(5.0f, 0.0f, 0.0f)); glm::vec4 newPosition = translationMatrix * glm::vec4(position, 1.0f); std::cout << “New Position: (“ << newPosition.x << “, “ << newPosition.y << “, “ << newPosition.z << “)\n”; return 0; } |
- Trigonometry: Trigonometry helps calculate angles, rotations, and movement in both 2D and 3D spaces. It’s critical for controlling the orientation of objects, such as rotating the camera or determining the trajectory of a projectile.
- Calculus: Calculus is essential for creating realistic motion and physics simulations. It helps model continuous changes in the game world, such as the acceleration of objects or fluid motion.
You can handle everything from physics simulations to camera controls and animations by learning these key mathematical concepts.
Step 5: Explore Graphics Programming
Graphics programming involves rendering a game’s visual elements, from models and textures to lighting effects and complex shaders. Understanding how to interact with graphics hardware through APIs is key to producing visually stunning games.
- Graphics APIs: APIs like OpenGL, DirectX, or Vulkan bridge the game and the graphics hardware. They allow you to control how 3D models are drawn, textures are applied, and visual effects like lighting and shadows are rendered.
- Shaders: Shaders are small programs that run on the GPU to render effects like lighting, textures, and post-processing. You can create custom visual effects and optimize rendering performance by learning shader languages like GLSL (for OpenGL) or HLSL (for DirectX).
Here’s an example of a simple vertex and fragment shader program in glsl.
Vertex Shader:
#version 330 core layout(location = 0) in vec3 aPos; void main() { gl_Position = vec4(aPos, 1.0); } |
Fragment Shader:
#version 330 core out vec4 FragColor; void main() { FragColor = vec4(1.0, 0.5, 0.2, 1.0); // Orange color } |
- Rendering Techniques: Rendering techniques determine how graphics are displayed on the screen. This includes handling lighting, shadows, reflections, and other visual effects.
Advanced techniques, such as ray tracing or global illumination, can create photorealistic visuals, while simpler techniques are used for less demanding projects.
Mastering graphics programming is essential for creating visually impressive games, especially in 3D, where rendering performance is a major concern.
Step 6: Specialize in Game Development Areas
Game development is a broad field with many areas of specialization. You can focus on specific areas, such as gameplay programming, AI, or graphics programming, depending on your interests.
- Gameplay Programming: This role is responsible for implementing the core mechanics of the game, such as player controls, character movement, and interaction with the game world. A strong understanding of game logic and flow is required to create smooth, engaging gameplay.
- AI Programming: AI is responsible for creating intelligent non-player characters (NPCs) that react to the player’s actions. Techniques like state machines, pathfinding algorithms (e.g., A*), and behavior trees are often used to create lifelike, responsive AI.
- Graphics Programming: This role focuses on making the game visually appealing, from creating realistic lighting and shaders to optimizing the rendering pipeline. It’s ideal for developers with a passion for computer graphics.
- Network Programming: Network programming ensures that multiplayer games work smoothly across the internet, managing player synchronization, lag compensation, and server communication.
Choosing a specialization allows you to deepen your expertise and contribute to specific aspects of game development.
Step 7: Practice with Game Projects
The best way to learn game development is by actually building games. Start with simple projects to practice the full development process, from game design and programming to testing and optimization.
- Start Small: Begin with small projects like basic 3D games. This helps you learn the fundamentals without getting overwhelmed by complexity.
- Expand Gradually: As you gain more experience, take on more complex projects. Experiment with different genres (platformers, puzzle games, etc.) to learn about various game mechanics and systems.
- Build a Portfolio: Create a portfolio of your work to showcase your skills. A strong portfolio is essential for attracting potential employers or clients.
Building games allows you to apply your knowledge, solve real-world problems, and showcase your creativity and skills.
Step 8: Stay Updated with Industry Trends
Game development is an ever-evolving field. Hence, staying updated with the latest tools, technologies, and best practices is essential to remain competitive.
- Follow Industry News: Regularly read game development blogs, news sites, and forums to keep track of the latest developments in game engines, graphics technologies, and game design.
- Engage with the Community: Join online communities like game development subreddits or Discord channels. These platforms are great for learning from others and staying informed about new trends.
- Attend Conferences and Meetups: Game development conferences like the Game Developers Conference (GDC) are valuable for networking, learning from industry leaders, and exploring new ideas.
Keeping up with the latest trends ensures you’re always aware of new tools and techniques to enhance your game development process.
Step 9: Build a Portfolio
A portfolio is a critical part of a game developer’s career. It showcases your skills and projects to potential employers or clients, making it easier for them to evaluate your abilities.
- Showcase Diverse Projects: Include various games demonstrating your skill range, from simple 2D games to more complex 3D projects.
- Highlight Key Skills: Emphasize the technical and creative skills you’ve developed, such as programming, game design, AI development, and graphics programming.
- Share Your Process: You should consider including breakdowns of your development process, such as how you approached certain challenges or implemented specific features.
A strong portfolio demonstrates your capabilities and helps you stand out in the competitive game development industry.
Step 10: Test and Debug Your Game
Testing and debugging are vital parts of game development. Games can contain bugs affecting gameplay, performance, or user experience, so thorough testing ensures the game works as intended.
- Automated Testing: Automated tests can be used to check for bugs, performance issues, and gameplay bugs before the game is released. Unit tests, integration tests, and regression tests all ensure the game’s stability.
- Manual Testing: Playtesting your game helps identify design flaws and balance issues. Manual testing can also uncover bugs that automated testing might miss.
- Debugging Tools: Tools like RenderDoc are great for debugging graphics-related issues, while profilers like NVIDIA Nsight and Unreal’s built-in profiler can be used to optimize game performance.
Thorough testing and debugging are essential to releasing a polished, playable game.
Winding Up
At Xpress Gaming, we are passionate about creating high-performance, immersive gaming experiences that captivate players. Our team of expert developers is skilled in C++, Unreal Engine, and other cutting-edge technologies, enabling us to craft games with precision and creativity.
Whether you’re aiming for AAA-quality graphics, intricate gameplay, or seamless cross-platform compatibility, we have the expertise to transform your game ideas into reality.
C++ is critical in 3D game development, offering the performance and control necessary for building high-end, scalable games. Its ability to efficiently manage memory, handle complex algorithms, and integrate with graphics APIs makes it the language of choice for top-tier game development.
Ready to create the next big hit? Connect with Xpress Gaming today, and let us bring your 3D game to life with the power of C++ and our advanced development expertise!
Client Testimonials Blog ID - 15559
At Kuriosity QA, we are committed to providing the highest quality gaming QA services to our clients in the gaming industry. Here’s what some of our satisfied clients have to say about working with us: